Qt Signal Slot Queued Connection
- In PyQt, connection between a signal and a slot can be achieved in different ways. Following are most commonly used techniques − QtCore.QObject.connect(widget, QtCore.SIGNAL(‘signalname’), slotfunction) A more convenient way to call a slotfunction, when a signal is emitted by a widget is as follows − widget.signal.connect(slotfunction).
- A developer can choose to connect to a signal by creating a function (a 'slot') and calling the connect function to relate the signal to the slot. Qt's signals and slots mechanism does not require classes to have knowledge of each other, which makes it much easier to develop highly reusable classes.
The second requirement is a consequence of the first: when a queued connection is activated, Qt needs to copy the arguments of the signal into the event sent to the receiver. In order to do so, it needs a bit of help from the user: all the types of the arguments must be registered in Qt’s meta-type system. A direct connection means that the slot is always invoked directly by the thread the signal is emitted from; a queued connection means that an event is posted in the event queue of the thread the receiver is living in, which will be picked up by the event loop and will cause the slot invocation sometime later.

Queued Connections
‹ Scoped Lock ● Producer Consumer Example ›
How to pass the produced soup to a consumer, e.g. to the main thread?
Passing messages between threads is easy with Qt. We simply pass objects through a signal/slot connection via so called queued connections.
Passing a message through a regular slot is done via parametrization:
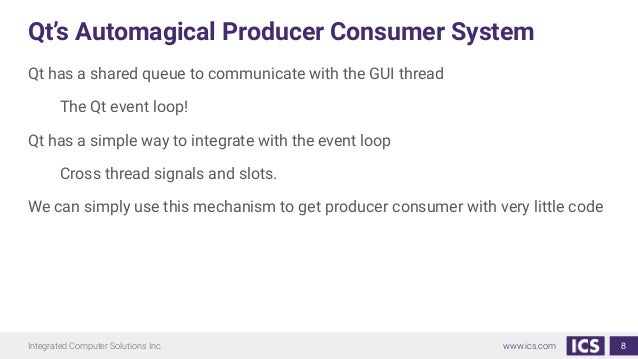
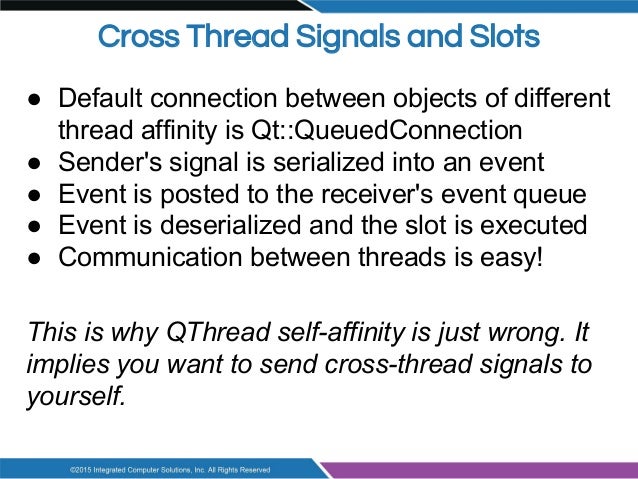
message sender:
message receiver:
Qt Signal Slot Queued Connection Tool
Then the receiver is invoked from the same thread as the sender.
To invoke the receiver on a different thread, we connect the signal and the slot with the option of a queued connection:
Qt Signal Slot Queuedconnection
receiver, SLOT(slot(QString &)),
Qt::QueuedConnection);

Then a triggered signal in the sender thread has the effect of a copy of the parameters being stored in the event queue. The sender returns immediately after the copy has been posted. The copy is delivered to the receiver when the receiver thread yields to the event loop.
This scheme works as long as
- The type of the passed parameters is a class with a copy constructor.
- Either the sender or the receiver have an event loop running.
- The type of the parameter is known to Qt.
If the data type is unknown we register it before connecting the respective signal:
‹ Scoped Lock ● Producer Consumer Example ›